PyScript is possible because of WASM. I wanted to see what is involved in calling functions located in a WASM module from Python. Turns out it is very easy to do at least at the function import and export level.
WASM is short for WebAssembly. WASM is a binary instruction format for a stack-based virtual machine. Wasm is designed as a portable compilation target for programming languages, enabling deployment on the web for client and server applications. [source]
The key component to PyScript is Pyodide. Pyodide is a WASM module about 9.5 MB in size. Python itself is written in C and for Pyodide the Python interpreter is compiled into WASM. As PyScript evolves, I envision that some parts will be delivered as separate WASM modules to reduce application load size. Knowing how to select and load these modules might be useful.
The primary benefits to WASM are execution speed and language independence. By targeting WASM, you can create applications in multiple languages. Popular languages today are C/C++, C#/Blazor, Go, and Rust. Commercial companies might be interested in delivering their technology via WASM as that is a form of obfuscation, making it several levels harder to reverse engineer. However, disassembly of WASM modules is easy, so this is not a bullet-proof protection method but it definitely is only for the most determined.
I will not be going in-depth into setting up and installing WASM tools. I will show how this is done at a higher level so that maybe you are motivated to learn more. I plan to write more in-depth articles as I find ways to integrate WASM and PyScript together.
I did find some very interesting tools and libraries for Python that provide amazing features for integrating WASM into Python programs that are not related to PyScript. One example is Wasmer which compiles and executes WASM inside a regular Python program. That is a great feature for development and testing.
Another important item is AssemblyScript. This is a typed language that is very close to Typescript/JavaScript.
The C Program
I wrote a very simple C program that provides one function: square(n)
.
1 2 3 4 |
int square(int n) { return n * n; } |
I compiled the C program using emscripten using this command line.
1 |
emcc -Os --no-entry -s EXPORTED_FUNCTIONS="['_square']" test.c -o test.wasm |
That command generates the file test.wasm
.
If you use Docker, which you should be, emscripten has a container with the compiler built-in. This command, for Windows, does not require any installation, except for downloading the container image.
1 2 3 4 5 6 |
set WORKDIR=%cd% docker run --rm ^ -v %WORKDIR%:/src ^ emscripten/emsdk ^ emcc -Os --no-entry -s EXPORTED_FUNCTIONS="['_square']" test.c -o test.wasm |
I wrote JavaScript to load the WASM module test.wasm
into memory.
1 2 3 4 5 6 7 8 9 10 |
<!-- Load WASM Module --> <script> WebAssembly.instantiateStreaming(fetch('test.wasm')) .then(obj => { wasm_exports = { square: obj.instance.exports.square }; } ); </script> |
The only thing different from the documentation that I did is to create a global JavaScript object called wasm_exports
. That object allows Python access to the WASM exports.
To call the WASM function square(n)
from Python is as easy as this:
1 2 3 |
from js import wasm_exports console.log('The square of 64 = ', wasm_exports.square(64)) |
Summary
Hopefully, this article will spark some interest and ideas for writing WASM modules in your favorite language and integrating them into your Python/PyScript programs. WASM is a complex topic with a large number of details and features. Understanding this technology might be important to your PyScript development. AL and ML are huge consumers of CPU cycles. Moving CPU-intensive functions into WASM can make your program significantly faster.
More Information
- Other articles that I have written on Pyscript
- Emscripten
- Emscripten Docker Image
- MDN: WebAssembly
- AssemblyScript
Photography Credit
I write free articles about technology. Recently, I learned about Pexels.com which provides free images. The image in this article is courtesy of Silvana Palacios at Pexels.
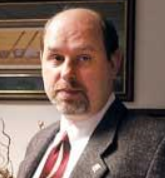
I design software for enterprise-class systems and data centers. My background is 30+ years in storage (SCSI, FC, iSCSI, disk arrays, imaging) virtualization. 20+ years in identity, security, and forensics.
For the past 14+ years, I have been working in the cloud (AWS, Azure, Google, Alibaba, IBM, Oracle) designing hybrid and multi-cloud software solutions. I am an MVP/GDE with several.
Leave a Reply